What is Stacked Area Plot?
Stacked area charts are extension of area charts which evaluates multiple groups in single chart. An area graph represents change in quantities of one or more groups over time. Using stackplot() function of matplotlib library to plot basic stacked area plot in python.
In this article, I will explain you about how to draw basic stacked area plot in python using various libraries like matplotlib, seaborn and Pandas
Using Parameters for Customizing Area Chart
Parameters using matplotlib.pyplot to customize stacked area plot are given below
Parameters ---------- x : 1d array of dimension N y : 2d array (dimension MxN), or sequence of 1d arrays (each dimension 1xN) The data is assumed to be unstacked. Each of the following calls is legal:: stackplot(x, y) # where y is MxN stackplot(x, y1, y2, y3, y4) # where y1, y2, y3, y4, are all 1xNm baseline : {'zero', 'sym', 'wiggle', 'weighted_wiggle'} Method used to calculate the baseline: - ``'zero'``: Constant zero baseline, i.e. a simple stacked plot. - ``'sym'``: Symmetric around zero and is sometimes called 'ThemeRiver'. - ``'wiggle'``: Minimizes the sum of the squared slopes. - ``'weighted_wiggle'``: Does the same but weights to account for size of each layer. It is also called 'Streamgraph'-layout. More details can be found at http://leebyron.com/streamgraph/. labels : Length N sequence of strings Labels to assign to each data series. colors : Length N sequence of colors A list or tuple of colors. These will be cycled through and used to colour the stacked areas. **kwargs All other keyword arguments are passed to `Axes.fill_between()`.
Parameters using pandas to customize area plot
Parameters ---------- x : label or position, optional Coordinates for the X axis. By default uses the index. y : label or position, optional Column to plot. By default uses all columns. stacked : bool, default True Area plots are stacked by default. Set to False to create a unstacked plot. **kwargs Additional keyword arguments are documented in :meth:`DataFrame.plot`.
Installation of Packages
We will need numpy, matplotlib and seaborn packages to plot customized stacked area chart in python. If you don’t have these packages installed on your system, install it using below commands.
pip install numpy pip install matplotlib pip install seaborn
Stacked Area Chart using Matplotlib
Let’s see an example to plot stacked area chart using matplotlib library input format.
Installation of Packages
We will need numpy and matplotlib packages to plot stacked area graph. Install packages using below command.
pip install numpy pip install matplotlib
Import libraries
Import numpy and matplotlib seaborn libraries in our python code to get started with plotting stacked area graph.
import numpy as np import matplotlib.pyplot as plt
Cool Tip: Learn How to plot bubble chart in python !
Prepare dataset
We will need x and y axis dataset values to plot stacked area chart. Let’s create dataset for x using Numpy arange() to get array object evenly spaces values within given range and for the 5 values of x we have assigned list of three list of 5 values representing three separate groups in y variable.
# Create Dataset for X and Y axis x=np.arange(1,6) y=[ [1,4,3,5,9], [2,3,9,6,10], [2,8,10,8,12] ]
Plot Stacked Area chart using stackplot()
The values of x and y dataset are assigned in stackplot(). We have assigned a list to lables parameter. We have assigned the location of legends as upper left in plot.
plt.stackplot(x,y, labels=['A','B','C']) plt.legend(loc='upper left')
Stacked Area plot Python Code
Use below source code for basic stacked area plot in python using Matplotlib
# Import libraries import numpy as np import matplotlib.pyplot as plt # --- FORMAT 1 # Create Dataset for X and Y axis x=np.arange(1,6) y=[ [1,4,3,5,9], [2,3,9,6,10], [2,8,10,8,12] ] # Basic stacked area chart. plt.stackplot(x,y, labels=['A','B','C']) plt.legend(loc='upper left')
Basic Stacked Area Plot Output
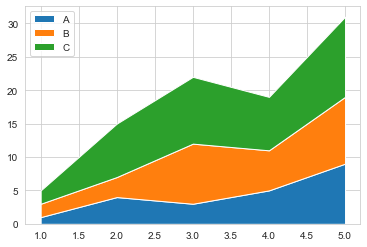
Cool Tip: Learn How to plot Pie chart in python !
Stacked Area Chart using Matplotlib
Let’s see an example to plot stacked area chart using matplotlib library input format.
Installation of Packages
We will need numpy and matplotlib packages to plot stacked area graph. Install packages using below command.
pip install numpy pip install matplotlib
Import libraries
Import numpy and matplotlib seaborn libraries in our python code to get started with plotting stacked area graph.
import numpy as np import matplotlib.pyplot as plt
Prepare dataset
We have created variables x and y1, y2, y3 as datasets to plot stacked area plot
We have assigned x as range of (1,6) which contain five values and for the 5 values of x, we have assigned list of 5 values representing separate groups as y1, y2, y3 variable.
# Create Dataset for X and Y axis x=np.arange(1,6) y1=[1,4,3,5,9] y2=[2,3,9,6,10] y3=[2,8,10,8,12]
Plot Stacked Area chart using stackplot()
The values of x and y1,y2 and y3 dataset are assigned in stackplot(). We have assigned a list to lables parameter. We have assigned the location of legends as upper left in plot.
# Basic stacked area chart. plt.stackplot(x,y1, y2, y3, labels=['A','B','C']) plt.legend(loc='upper left')
Stacked Area plot Python Code
Use below source code for basic stacked area plot in python using Matplotlib
# Import libraries import numpy as np import matplotlib.pyplot as plt # Create Dataset for X and Y axis x=np.arange(1,6) y1=[1,4,3,5,9] y2=[2,3,9,6,10] y3=[2,8,10,8,12] # Basic stacked area chart. plt.stackplot(x,y1, y2, y3, labels=['A','B','C']) plt.legend(loc='upper left')
Basic Stacked Area Plot Output
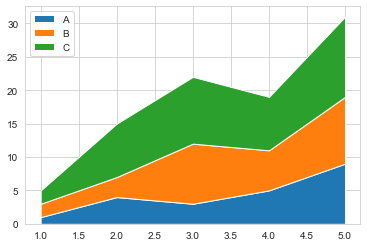
Cool Tip: Learn How to plot Treemap Chart in python !
Stacked Area Chart using Seaborn
Let’s see an example to plot stacked area chart using matplotlib and seaborn library input format.
Installation of Packages
We will need numpy, matplotlib and seaborn packages to plot stacked area graph. Install packages using below command.
pip install numpy pip install matplotlib pip install seaborn
Import libraries
Import numpy, matplotlib seaborn libraries in our python code to get started with plotting stacked area graph.
import numpy as np import matplotlib.pyplot as plt import seaborn as sns
Prepare dataset
We have created variables x and y as datasets to plot stacked area plot
We have assigned x as range of (1,6) which contain five values and for the 5 values of x, we have assigned list of 5 values representing separate groups as y1, y2, y3 variable.
# Create Dataset for X and Y axis x=np.arange(1,6) y=[ [1,4,3,5,9], [2,3,9,6,10], [2,8,10,8,12] ] pal = sns.color_palette("Set1")
Plot Stacked Area chart using stackplot()
The values of x and y dataset are assigned in stackplot(). We have assigned a list to lables parameter. We have assigned the location of legends as upper left in plot.
# Basic stacked area chart. plt.stackplot(x,y, labels=['A','B','C']) plt.legend(loc='upper left')
Show Basic Stacked Area Graph
Using Matplotlib show() function to show the graphical view of area chart.
plt.show()
Stacked Area plot Python Code
Use below source code for basic stacked area plot in python using Matplotlib
# library import numpy as np import matplotlib.pyplot as plt import seaborn as sns # Create Dataset for X and Y axis x=np.arange(1,6) y=[ [1,4,3,5,9], [2,3,9,6,10], [2,8,10,8,12] ] pal = sns.color_palette("Set1") # Plot plt.stackplot(x,y, labels=['A','B','C'],colors=pal) plt.legend(loc='upper left') plt.show()
Show Basic Area Stacked Chart
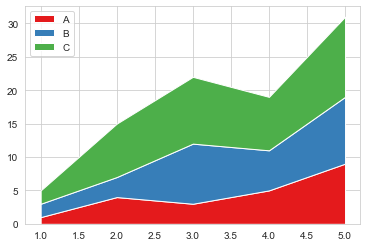
Stacked Area Chart using Pandas
Let’s see an example to plot stacked area chart using pandas library.
Installation of Packages
We will need numpy, matplotlib and pandas packages to plot stacked area plot. Install packages using below command.
pip install numpy pip install matplotlib pip install pandas
Import libraries
Import numpy, matplotlib and pandas libraries in our python code to get started with plotting stacked area graph.
import numpy as np import matplotlib.pyplot as plt import pandas as pd
Prepare dataset
We have created a dataset of 15 rows and 4 columns using dataframe and random.rand function. We have also declared a list to the columns parameter.
df = pd.DataFrame(np.random.rand(15, 4), columns=['a', 'b', 'c', 'd'])
Plot Stacked Area chart using Pandas Plot
We have used area function of plot in pandas library to plot stacked area plot.
df.plot.area()
Basic Stacked Area Plot Source Code
Use below entire source code for basic stacked area plot in python using Pandas library.
# Import libraries import pandas as pd import numpy as np import matplotlib.pyplot as plt # Dataset df = pd.DataFrame(np.random.rand(15, 4), columns=['a', 'b', 'c', 'd']) # plot df.plot.area()
Basic Stacked Area Plot using Pandas Output
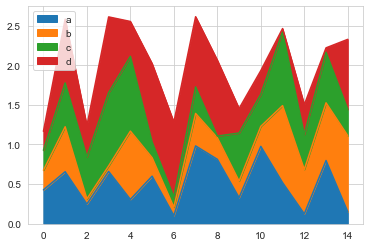
Cool Tip: Learn How to create Q-Q Plot in python !
Conclusion
I hope you found above article on basic stacked area plot in python using matplotlib ,seaborn and Pandas package informative and educational.