In this article, I will explain you how to control bandwidth of density plot in python with larger bandwidth and narrow bandwidth methods. Density plot require numeric values dataset to display plot in python.
To control bandwidth of density plot in python use kdeplot()
function of seaborn library with bw_adjust
or bw_method
parameter
Installation of Packages
We will need seaborn package to load dataset and plot density graph in python program. If you don’t have these packages installed on your system, install it using below commands.
pip install seaborn
Cool Tip: Know more about how to plot vertical subplot line graph in python!
Control Bandwidth of Density Plot in Python (Large Bandwidth)
Let’s see an example to control bandwidth of density plot as large bandwidth in python using seaborn library.
Installation of Packages
We will need seaborn package to show density plot. Install package using below command.
pip install seaborn
Import libraries
Import seaborn library in our python density plot code.
# Import library import seaborn as sns
Prepare dataset
Seaborn library has inbuilt datasets. We will use tips dataset to visualize density plot
- Load tips dataset using load_dataset() function of seaborn library in df variable
# Load the tips dataset df = sns.load_dataset('tips')
Plot Density Graph using kdeplot()
Use sns.kdeplot()
function of seaborn module to draw density graph.
- The first argument in kdeplot() defines dataset use for plot
- The second argument in kdeplot() defines shade as True
- The third argument in kdeplot() defines bw_adjust = 5 to control bandwith. You can use either bw_adjust or bw_method
- The fourth argument in kdeplot() define color
# Plot Density Graph with large bandwidth sns.kdeplot(df['total_bill'], shade=True, bw_adjust=5, color="olive")
Density Plot with large bandwidth Python Code
Use below entire density graph python code using seaborn library
# library & dataset import seaborn as sns df = sns.load_dataset('tips') # Plot Density Graph with large bandwidth sns.kdeplot(df['total_bill'], shade=True, bw_adjust=5, color="olive")
Density Plot with large bandwidth Python Visualization Output
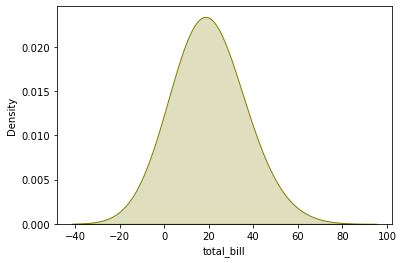
Cool Tip: Know more about how to plot marginal histogram plot in python!
Control Bandwidth of Density Plot in Python (Narrow Bandwidth)
Let’s see an example to plot density plot with narrow bandwidth in python with shade.
Installation of Packages
We will need seaborn package to plot density graph. Install package using below command.
pip install seaborn
Import libraries
Import seaborn library in our python density plot code.
# Import library import seaborn as sns
Prepare dataset
Seaborn library has inbuilt datasets. We will use tips dataset to visualize density graph
- Load tips dataset using load_dataset() function of seaborn library in df variable
# Load the tips dataset df = sns.load_dataset('tips')
Plot Density Graph using kdeplot()
Use sns.kdeplot()
function of seaborn module to draw density plot with shade.
- The first argument in kdeplot() defines dataset use to plot density.
- The second argument in kdeplot() defines shade = True
- The third argument in kdeplot() defines bw_adjust=0.5 to control bandwidth as narrow.
- The fourth argument in kdeplot() function defines color as orange
# Plot Density Graph with large bandwidth sns.kdeplot(df['total_bill'], shade=True, bw_adjust=0.5, color="orange")
Cool Tip: Know more about how to plot parallel coordinates graph in python!
Density Plot with Narrow Bandwidth Python Code
Use below entire density plot python code using seaborn library
# Import library import seaborn as sns # Load the tips dataset df = sns.load_dataset('tips') # Plot Density Graph with large bandwidth sns.kdeplot(df['total_bill'], shade=True, bw_adjust=0.5, color="orange")
Python Density Plot with Narrow Bandwidth Visualization Output
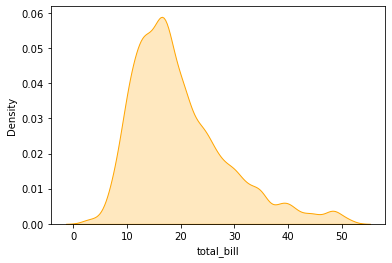
Cool Tip: Know more about how to plot bubble chart in python!
Conclusion
I hope you found above article on control bandwidth of density plot in python program using seaborn package informative and educational.
Use sns.kdeplot()
function of seaborn module to create density plot in python program. Using bw-adjust or bw_method to control bandwidth of plot as large bandwidth or narrow bandwidth.