Use the function kdeplot() of the seaborn library to draw a density plot in Python.
In this article, we will discuss how to plot multiple variables on a density plot like two variables density plot and three variables density plot.
Density plots require a numeric values dataset to display the plot in python.
Installation of Packages
We will need a seaborn package to load the dataset and plot the density graph in the python program. If you don’t have these packages installed on your system, install them using the below commands.
pip install seaborn
Cool Tip: Know more about how to plot histogram with several variables in python!
Plot Multiple Variables in Density Plot
Let’s see an example to plot two variable density plot in a single axis in python using the seaborn library.
Installation of Packages
We will need a seaborn package to show the density plot. Install the package using the below command.
pip install seaborn
Import libraries
Import the seaborn library in our python density plot code.
# Import library import seaborn as sns
Prepare dataset
Seaborn library has inbuilt datasets. We will use the tips
dataset to visualize the density plot
- Load tips dataset using
load_dataset()
function of the seaborn library in the df variable - We will use the
total_bill
andtip
column from the tips dataset.
# Load the tips dataset df = sns.load_dataset('tips')
Plot Density Graph using kdeplot()
Use sns.kdeplot() function of the seaborn module to draw a density graph.
- The first argument in kdeplot() defines the dataset to use for the plot.
- The second argument defines shade as true.
- The third argument defines color as red for the
total_bill
column and blue color for thetip
column dataset.
# Plot 2 variables on Density Graph p1=sns.kdeplot(df['total_bill'], shade=True, color="r") p1=sns.kdeplot(df['tip'], shade=True, color="b")
Two Variables on Density Plot Python Code
Use entire density python code below using the seaborn library
# library and dataset import seaborn as sns df = sns.load_dataset('tips') # plot of 2 variables on Density Graph p1=sns.kdeplot(df['total_bill'], shade=True, color="r") p1=sns.kdeplot(df['tip'], shade=True, color="b")
Two Variables on Density Plot Python Visualization Output
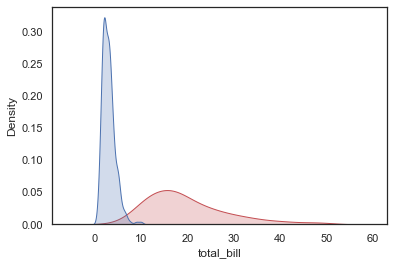
Cool Tip: Know more about how to plot customized line graphs in python!
Plot Three Variables in Density Plot
Let’s see an example to plot three variable density plot in a single axis in python using the seaborn library.
Installation of Packages
We will need a seaborn package to show the density plot. Install the package using the below command.
pip install seaborn
Import libraries
Import the seaborn library in our python density plot code.
# Import library import seaborn as sns
Prepare dataset
Seaborn library has inbuilt datasets. We will use the penguins
dataset to visualize the density plot
- Load tips dataset using load_dataset() function of the seaborn library in df variable
- We will use bill_length_mm, bill_depth_mm, and flipper_length_mm columns from the
penguins
dataset.
# Load the tips dataset df = sns.load_dataset('penguins')
Plot Density Graph using kdeplot()
Use the sns.kdeplot() function of the seaborn module to draw a density graph.
- The first argument
kdeplot()
defines the dataset to use for the plot - The second argument defines shade as
true
- The third argument defines color as red for the
total_bill
column and blue color for thetip
column dataset.
# plot 3 variables on density graph p1=sns.kdeplot(df['bill_length_mm'], shade=True, color="r") p1=sns.kdeplot(df['bill_depth_mm'], shade=True, color="b") p1=sns.kdeplot(df['flipper_length_mm'], shade=True, color="g")
Three Variables on Density Plot Python Code
Use entire density python code below using the seaborn library
# library and dataset import seaborn as sns df = sns.load_dataset('penguins') # plot 3 variables on density graph p1=sns.kdeplot(df['bill_length_mm'], shade=True, color="r") p1=sns.kdeplot(df['bill_depth_mm'], shade=True, color="b") p1=sns.kdeplot(df['flipper_length_mm'], shade=True, color="g")
Three Variables on Density Plot Python Visualization Output
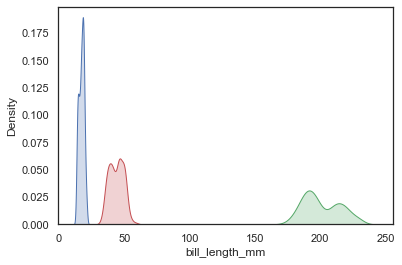
Cool Tip: Know more about how to plot customized stacked area graphs in python!
Conclusion
I hope you found the above article to plot multiple variables on density plot in a python program using the seaborn package informative and educational.
Use the sns.kdeplot() function of the seaborn module to create a density plot in the python program.