use plot() and subplot()
function of matplotlib library to plot multiple subplot line chart in python using matplotlib package,
In this article, I will explain you how to plot multiple subplot line chart in python using matplotlib package.
Installation of Packages
We will need numpy and matplotlib packages to create multiple subplot line chart in python. If you don’t have these packages installed on your system, install it using below commands.
pip install matplotlib pip install numpy
Parameters for Customization of Line Plot
using below parameters in plot() function to customized line plot with line style, color and marker.
plot(x, y) # plot x and y using default line style and color plot(x, y, 'bo') # plot x and y using blue circle markers plot(y) # plot y using x as index array 0..N-1 plot(y, 'r+') # ditto, but with red plusses representation formats for styling **Markers** ============= =============================== character description ============= =============================== ``'.'`` point marker ``','`` pixel marker ``'o'`` circle marker ``'v'`` triangle down marker ``'^'`` triangle up marker ``'<'`` triangle left marker ``'>'`` triangle right marker ``'1'`` tri down marker ``'2'`` tri_up marker ``'3'`` tri_left marker ``'4'`` tri_right marker ``'s'`` square marker ``'p'`` pentagon marker ``'*'`` star marker ``'h'`` hexagon1 marker ``'H'`` hexagon2 marker ``'+'`` plus marker ``'x'`` x marker ``'D'`` diamond marker ``'d'`` thin_diamond marker ``'|'`` vline marker ``'_'`` hline marker ============= =============================== **Line Styles** ============= =============================== character description ============= =============================== ``'-'`` solid line style ``'--'`` dashed line style ``'-.'`` dash-dot line style ``':'`` dotted line style ============= =============================== Example format strings:: 'b' # blue markers with default shape 'or' # red circles '-g' # green solid line '--' # dashed line with default color '^k:' # black triangle_up markers connected by a dotted line **Colors** The supported color abbreviations are the single letter codes ============= =============================== character color ============= =============================== ``'b'`` blue ``'g'`` green ``'r'`` red ``'c'`` cyan ``'m'`` magenta ``'y'`` yellow ``'k'`` black ``'w'`` white ============= =============================== We can use any combination of color, markers and styling to the graph. And make the graph customized the way we want.
How to Plot Multiple Subplot Line Graph in Python
Let’s see an example to plot multiple subplot line chart using matplotlib library
Installation of Packages
We will need numpy and matplotlib packages to plot line graph. Install packages using below command.
pip install matplotlib pip install numpy
Import libraries
Import numpy and matplotlib libraries in our python multiple line graph code to get started with plotting line chart.
# Import library import matplotlib.pyplot as plt import numpy as np
Prepare dataset
Create a dataset for x and y axis to plot multiple subplot line chart to display 4 subplot line graph
- Prepare dataset for x axis
- Create dataset for y axis
# Prepare for datasets x=np.arange(1,5) y=x**3
Draw Multiple subplots Line chart using Plot()
Use plt.subplot()
function of matplotlib module to multiple subplots.
We have used tuple in subplot(). tuple format is (rows, columns, number of plot)
In below code, create 4 subplot each having subplot() function to accept tuple, plot() function to plot line graph with black color with dotted line and subplot line chart title.
# Subplot 1 plt.subplot(2,2,1) plt.plot([1,2,3,4],[1,4,9,16],'k*:') plt.title('1st plot') # Subplot 2 plt.subplot(2,2,2) plt.plot(x,y,'rs-.') plt.title('2nd plot') # Subplot 3 plt.subplot(2,2,3) plt.plot(x+10,y-10,'bp--') plt.title('3rd plot') # Subplot 4 plt.subplot(2,2,4) plt.plot(x,y+3,'yo-') plt.title('4th plot')
Plot Multiple Subplot Line Chart
Use show() function of matplotlib.pyplot module to plot line graph
# Set title plt.suptitle('Multiple Subplots') plt.show()
Python Multiple Subplot Line Plot Code
Use below entire multiple subplots Line chart in python code using matplotlib library
# Import library import matplotlib.pyplot as plt import numpy as np # Prepare for datasets x=np.arange(1,5) y=x**3 # Subplot 1 plt.subplot(2,2,1) plt.plot([1,2,3,4],[1,4,9,16],'k*:') plt.title('1st plot') # Subplot 2 plt.subplot(2,2,2) plt.plot(x,y,'rs-.') plt.title('2nd plot') # Subplot 3 plt.subplot(2,2,3) plt.plot(x+10,y-10,'bp--') plt.title('3rd plot') # Subplot 4 plt.subplot(2,2,4) plt.plot(x,y+3,'yo-') plt.title('4th plot') # Set title plt.suptitle('Multiple Subplots') plt.show()
Multiple Subplot Line Chart Visualization Output
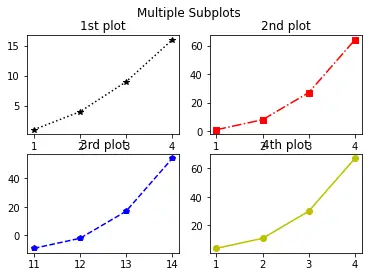
Cool Tip: Learn How to plot horizontal line graph in python !
Conclusion
I hope you found above article on Multiple Subplot Line Graph in Python using matplotlib informative and educational.
Use plt.plot()
and plt.subplot()
function of matplotlib module to create multiple subplot line chart.