What is Line Plot?
Line graph is basically a graph of a line joining the data points. Data points are also known as markers. The graph can be of any shape. For ex. straight line, curve, or any other shape. Line plot is a basic type of chart which is commonly used in many fields. To plot horizontal line graph in python using matplotlib package, use plot() function of matplotlib library.
In this article, I will explain you how to plot horizontal line graph in python using matplotlib package.
Installation of Packages
We will need matplotlib packages to create horizontal line chart in python. If you don’t have these packages installed on your system, install it using below commands.
pip install matplotlib
Parameters for Customization of Line Plot
plot(x, y) # plot x and y using default line style and color plot(x, y, 'bo') # plot x and y using blue circle markers plot(y) # plot y using x as index array 0..N-1 plot(y, 'r+') # ditto, but with red plusses representation formats for styling **Markers** ============= =============================== character description ============= =============================== ``'.'`` point marker ``','`` pixel marker ``'o'`` circle marker ``'v'`` triangle down marker ``'^'`` triangle up marker ``'<'`` triangle left marker ``'>'`` triangle right marker ``'1'`` tri down marker ``'2'`` tri_up marker ``'3'`` tri_left marker ``'4'`` tri_right marker ``'s'`` square marker ``'p'`` pentagon marker ``'*'`` star marker ``'h'`` hexagon1 marker ``'H'`` hexagon2 marker ``'+'`` plus marker ``'x'`` x marker ``'D'`` diamond marker ``'d'`` thin_diamond marker ``'|'`` vline marker ``'_'`` hline marker ============= =============================== **Line Styles** ============= =============================== character description ============= =============================== ``'-'`` solid line style ``'--'`` dashed line style ``'-.'`` dash-dot line style ``':'`` dotted line style ============= =============================== Example format strings:: 'b' # blue markers with default shape 'or' # red circles '-g' # green solid line '--' # dashed line with default color '^k:' # black triangle_up markers connected by a dotted line **Colors** The supported color abbreviations are the single letter codes ============= =============================== character color ============= =============================== ``'b'`` blue ``'g'`` green ``'r'`` red ``'c'`` cyan ``'m'`` magenta ``'y'`` yellow ``'k'`` black ``'w'`` white ============= =============================== We can use any combination of color, markers and styling to the graph. And make the graph customized the way we want.
How to Plot Horizontal Line Graph in Python
Let’s see an example to plot horizontal line chart using matplotlib library
Installation of Packages
We will need matplotlib packages to show line graph in python. Install packages using below command.
pip install matplotlib
Import libraries
Import matplotlib libraries in our python horizontal line graph code to get started with plotting line chart.
# Import library import matplotlib.pyplot as plt
Cool Tip: Learn How to plot customized line chart in python !
Prepare dataset
Create a dataset for x and y axis to plot horizontal line chart in python to display air quality forecast for week
- Prepare dataset of days for x axis
- Create dataset of temperature for y axis
# Prepare dataset for x and y axis angle=(0,30,45,60,90,120,135,150,180) sine=(0,1/2,(2**0.5)/2,(3**0.5)/2,1,(3**0.5)/2,(2**0.5)/2,1/2,0) cos=(1,(3**0.5)/2,(2**0.5)/2,1/2,0,-1/2,-(2**0.5)/2,-(3**0.5)/2,-1)
Draw Horizontal Line subplot using Plot()
Use plt.subplot()
function of matplotlib module to draw line graph.
Use matplotlib title and label function to assign title and label for x axis and y axis.
We have used tuple in subplot(). tuple format is (rows, columns, number of plot)
In subplot 1, we have plotted sine wave.
# Plot sublot 1 for sine function plt.subplot(2,1,1) plt.plot(angle,sine,'ro-') # Set the title and Labels plt.title('sine function') plt.xlabel('angle') plt.ylabel('sine')
In subplot 2, we have plotted cosine wave.
# Plot sublot 1 for cosine function plt.subplot(2,1,2) plt.plot(angle,cos,'bo-') # Set the title and Labels plt.title('cos function') plt.xlabel('angle') plt.ylabel('cos')
Plot Horizontal Line Graph
Use show() function of matplotlib.pyplot module to plot line graph
# set title plt.suptitle('Trigonomatric function') plt.show()
Python Horizontal Line Plot Code
Use below entire horizontal Line plot in python code using matplotlib library
# Import library import matplotlib.pyplot as plt # Prepare Datasets angle=(0,30,45,60,90,120,135,150,180) sine=(0,1/2,(2**0.5)/2,(3**0.5)/2,1,(3**0.5)/2,(2**0.5)/2,1/2,0) cos=(1,(3**0.5)/2,(2**0.5)/2,1/2,0,-1/2,-(2**0.5)/2,-(3**0.5)/2,-1) # Plot sublot 1 for sine function plt.subplot(2,1,1) plt.plot(angle,sine,'ro-') plt.title('sine function') # Plot sublot 1 for cosine function plt.subplot(2,1,2) plt.plot(angle,cos,'bo-') plt.title('cos function',loc='right') # Set title plt.suptitle('Trigonomatric function') plt.show()
Horizontal Line Graph Output
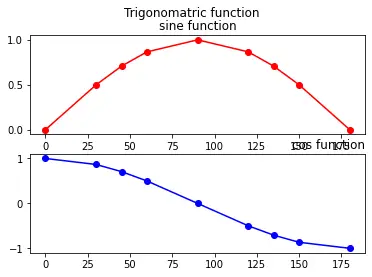
Conclusion
I hope you found above article on horizontal line chart in python using matplotlib and numpy package informative and educational.
Use plt.plot()
function of matplotlib module to create line chart.