In this article, I will explain you how to control rug and density of histogram plot using Python in four different ways.
Let’s understand each method with an example
To plot histogram in python use displot() function of seaborn library and control rug and density appearance using rug and kde parameters
Installation of Packages
We will need seaborn packages to load dataset and plot histogram If you don’t have these packages installed on your system, install it using below commands.
pip install seaborn
How to Plot Histogram without Rug
Let’s see an example to plot histogram plot in python with rug set to false.
Installation of Packages
We will need seaborn packages to show histogram. Install package using below command.
pip install seaborn
Import libraries
Import seaborn library in our python histogram code.
# Import library import seaborn as sns
Prepare dataset
Seaborn library has inbuilt datasets. We will use tips dataset to visualize histogram
- Load tips dataset using load_dataset() function of seaborn library
# Load the tips dataset df = sns.load_dataset('tips')
Cool Tip: Learn How to plot line graph in python !
Plot Histogram using jointplot()
Use sns.displot()
function of seaborn module to draw histogram
- The first argument defines data for histogram from tips dataset
- The second argument defines kind as hist
- The third argument defines kde set as false
- The fourth argument defines
rug
set as False
# Plot Histogram plot sns.displot(data= df["total_bill"],kind="hist",kde=False, rug=False )
Histogram Chart Python Code
Use below entire histogram python code using seaborn library
# Import library and dataset import seaborn as sns df = sns.load_dataset('tips') # Plot Histogram sns.displot(data= df["total_bill"],kind="hist",kde=False, rug=False )
Histogram Python Visualization Output
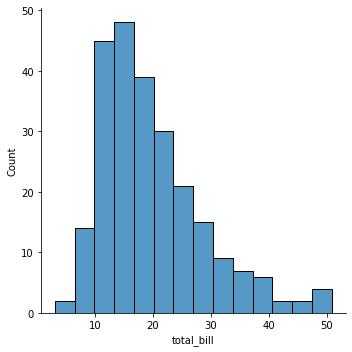
Cool Tip: Learn How to plot vertical subplot line graph in python !
Histogram Plot with Rug and Kernel Density
Let’s see an example to plot histogram with rug and kernel density in Python
Installation of Packages
We will need seaborn package to load dataset and plot histogram. Install package using below command.
pip install matplotlib
Import libraries
Import seaborn library in our python histogram code.
# Import library import seaborn as sns
Prepare dataset
Seaborn library has inbuilt datasets. We will use tips dataset to visualize histogram
- Load tips dataset using load_dataset() function of seaborn library in variable df
# Load the tips dataset df = sns.load_dataset('tips')
Plot Histogram with Rug and Density
- Use displot() function to plot histogram
- The first argument defines data for histogram from seaborn library tips dataset
- The second argument defines kind set as hist
- The third argument defines kde set as True
- The fourth argument defines rug set as True
- Appearance of rug and kernel density in histogram can be set using True or False
# Histogram + Rug + kernel density sns.displot(data= df["total_bill"],kind="hist",kde=True, rug=True )
Histogram with rug and kernel density Python Code
Use below entire histogram python code using seaborn library
# Import library and dataset import seaborn as sns df = sns.load_dataset('tips') # Histogram + Rug + kernel density sns.displot(data= df["total_bill"],kind="hist",kde=True, rug=True )
Python Histogram Plot with Rug and Kernel Density Visualization Output
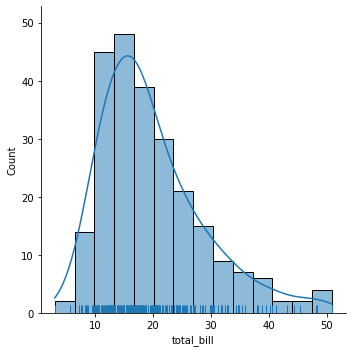
Cool Tip: Learn How to plot Treemap chart in python !
Plot Python Histogram with Customized Density
Let’s see an example to plot histogram with kernel density customization in python.
Installation of Packages
We will need seaborn package to load tips dataset and plot histogram. Install package using below command.
pip install seaborn
Import libraries
Import seaborn library in our python histogram code.
# Import library import seaborn as sns
Prepare dataset
Seaborn library has inbuilt datasets. We will use tips dataset to visualize histogram
- Load tips dataset using load_dataset() function of seaborn library
# Load the tips dataset df = sns.load_dataset('tips')
Plot Histogram using histplot()
Use sns.distplot()
function of seaborn module to draw histogram.
- The first argument defines dataset for histogram
- The second argument defines kernel density (kde) set as True
- The third argument defines parameters for density distribution as color = green, line width , transparency set as 0.3
# To change parameters of density distribution sns.distplot( a=df["total_bill"],kde=True,kde_kws={"color": "g", "alpha":0.3, "linewidth": 5, "shade":True })
Python Histogram with Kernel Density Code
Use below entire python histogram code using seaborn library
# Import library and dataset import seaborn as sns df = sns.load_dataset('tips') # To change parameters of density distribution sns.distplot( a=df["total_bill"],kde=True,kde_kws={"color": "g", "alpha":0.3, "linewidth": 5, "shade":True })
Python Histogram with kernel density Visualization Output
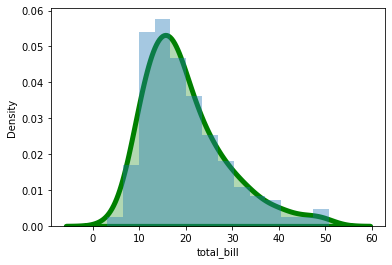
Cool Tip: Learn How to plot horizontal line graph in python !
Plot Python Histogram with Rug Control
Let’s see an example to plot histogram with rug customization in python.
Installation of Packages
We will need seaborn package to load tips dataset and plot histogram. Install package using below command.
pip install seaborn
Import libraries
Import seaborn library in our python histogram code.
# Import library import seaborn as sns
Prepare dataset
Seaborn library has inbuilt datasets. We will use tips dataset to visualize histogram
- Load tips dataset using load_dataset() function of seaborn library
# Load the tips dataset df = sns.load_dataset('tips')
Plot Histogram using histplot()
Use sns.distplot()
function of seaborn module to draw histogram.
- The first argument defines dataset for histogram
- The second argument defines rug set as True
- The third argument defines parameters for rug as color = re, line width , transparency set as 0.3
# To change parameters of rug sns.distplot( df["total_bill"], rug=True,rug_kws={"color": "r", "alpha":0.3, "linewidth": 2, "height":0.2 })
Python Histogram with rug customization Code
Use below entire python histogram code using seaborn library
# Import library and dataset import seaborn as sns df = sns.load_dataset('tips') # To change parameters of rug sns.distplot( df["total_bill"], rug=True,rug_kws={"color": "r", "alpha":0.3, "linewidth": 2, "height":0.2 })
Python Histogram with rug Visualization Output
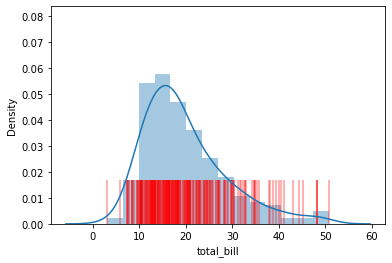
Conclusion
I hope you found above article on histogram with rug and density customization in python program using seaborn package informative and educational.
Use sns.displot()
function of seaborn module to create histogram in python program and rug and kde parameter to control appearance of rug and density