What is Treemap Chart?
Treemap are alternative way to display tree diagrams. Treemap plots maps hierarchical structure for categories given. It also shows the value of each category. To plot treemap chart in python, use squarify.plot() function of Squarify package.
In this article, I will explain you how to plot treemap chart in python using matplotlib and squarify package with examples on treemap visualization.
Installation of Packages
We will need matplotlib, pandas and squarify packages to plot treemap chart in python. If you don’t have these packages installed on your system, install it using below commands.
pip install matplotlib pip install numpy
Parameters for Customization of Treemap Chart
Parameters ---------- sizes input for squarify norm_x, norm_y x and y values for normalization color color string or list-like (see Matplotlib documentation for details) label list-like used as label text value list-like used as value text (in most cases identical with sizes argument) ax Matplotlib Axes instance pad draw rectangles with a small gap between them bar_kwargs : dict keyword arguments passed to matplotlib.Axes.bar text_kwargs : dict keyword arguments passed to matplotlib.Axes.text **kwargs Any additional kwargs are merged into `bar_kwargs`. Explicitly provided kwargs here will take precedence.
How to Plot Treemap Chart in Python
Let’s see an treemap chart example using squarify library
Installation of Packages
We will need pandas, matplotlib and squarify libraries to show treemap graph. Install packages using below command.
pip install matplotlib pip install pandas pip install squarify
Import libraries
Import pandas, matplotlib.pyplot and squarify libraries in our python treemap chart code.
# Import libraries import matplotlib.pyplot as plt import pandas as pd import squarify as
Prepare dataset
Create a dataframe using pandas to plot treemap chart in python. It constructs dataframe from dictionary using dataframe function of pandas module.
- The first argument defines size in the dictionary
- The second argument defines the groups in the dictionary
# Prepare for datasets df = pd.DataFrame({'size':[20,45,33,90], 'group':["Microsoft", "Facebook", "Google", "Tesla"] })
Cool Tip: Learn How to plot basic area plot in python !
Plot Treemap Chart using Plot()
Use sqarify.plot()
function of squarify module to plot treemap chart.
- The first argument defines size from the dataframe
- The second argument defines label
- The third argument defines transparency
# Plot Treemap Chart squarify.plot(sizes=df['size'], label=df['group'], alpha=.8 )
Show Treemap Chart for visualization
Use show() function of matplotlib.pyplot module to plot treemap chart
# Show Treemap Chart plt.axis('off') plt.show()
Treemap Python Code
Use below entire treemap chart python code
# Import libraries import matplotlib.pyplot as plt import pandas as pd import squarify # Prepare for datasets df = pd.DataFrame({'size':[20,45,33,90], 'group':["Microsoft", "Facebook", "Google", "Tesla"] }) # Plot Treemap Chart squarify.plot(sizes=df['size'], label=df['group'], alpha=.8 ) # Show Treemap Chart plt.axis('off') plt.show()
Python Treemap Visualization Output
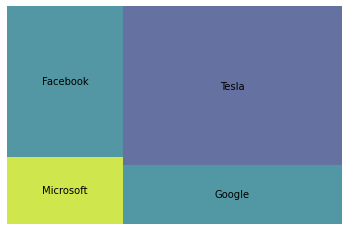
Cool Tip: Learn How to plot bubble chart in python !
Conclusion
I hope you found above Treemap Chart in Python tutorial with examples informative and educational.
Use squarify.plot()
function of squarify module to plot and visualize treemap chart.